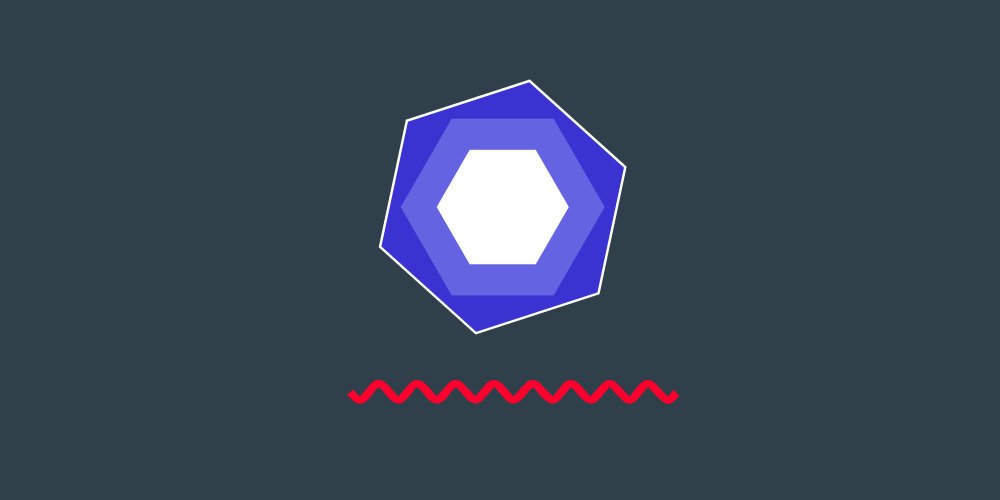
Eslint. How to set up in the right way
Eslint
can help you avoid standard JavaScript
errors through static code analysis.
Install the latest version of Eslint
, additional packages with configurations and the plugin for Prettier
autoformating tool.
npm i -D eslint \
eslint-config-airbnb-base \
eslint-config-prettier \
eslint-plugin-prettier
This is the configuration that I use on many projects, and it is subjective, you can add your configurations as desired.
For Drupal
theme, configuration files are different because we are inheriting the Eslint
configuration from the Drupal
core configuration.
npm i -D eslint \
eslint-config-airbnb \
eslint-config-prettier \
eslint-plugin-import \
eslint-plugin-jsx-a11y \
eslint-plugin-prettier \
eslint-plugin-react
Ignoring 3rd party libraries
The developer does not need to check the code of 3rd party libraries or compiled production code. Disabling unnecessary checks will save the resources of the IDE.
You can use the .eslintignore
file to set up ignoring rules.
An example of my .eslintignore
file:
node_modules/**
vendor/**
dist/**
The syntax is simple and similar to that of .gitignore
.
Ignore files or individual lines
This is not recommended, but sometimes it is still necessary to add a line or whole file ignore marker (useful for refactoring).
Ignoring an entire JS file:
// eslint-ignore
or
// eslint-disable
Ignoring a line or code fragment:
// eslint-disable
console.log('hi')
// eslint-enable
// eslint-disable-next-line
console.log('hi')
Ignoring a certain rule:
// eslint-disable no-console
// eslint-disable-next-line no-console
console.log('hi')
// eslint-disable no-console
console.log('hi')
// eslint-enable no-console
Integration with Prettier
To integrate Eslint
with Prettier, add the following line to the .eslintrc.json
configuration file:
{
"plugins": ["prettier"]
}
Examples of configuration files
Eslint for a static site
The configuration file I use for static sites:
{
"extends": ["airbnb-base", "plugin:prettier/recommended"],
"root": true,
"env": {
"browser": true,
"es6": true,
"node": true
},
"globals": {},
"plugins": ["prettier"],
"rules": {
"prettier/prettier": "error",
"consistent-return": ["off"],
"no-underscore-dangle": ["off"],
"max-nested-callbacks": ["warn", 3],
"import/no-mutable-exports": ["warn"],
"import/extensions": ["off"],
"import/no-extraneous-dependencies": [
"error",
{
"devDependencies": true
}
],
"no-plusplus": [
"warn",
{
"allowForLoopAfterthoughts": true
}
],
"no-param-reassign": ["off"],
"no-prototype-builtins": ["off"],
"valid-jsdoc": [
"warn",
{
"prefer": {
"returns": "return",
"property": "prop"
},
"requireReturn": false
}
],
"no-unused-vars": ["warn"],
"operator-linebreak": ["error", "after", { "overrides": { "?": "ignore", ":": "ignore" } }],
"quotes": ["error", "single"],
"comma-dangle": [
"error",
{
"arrays": "always-multiline",
"objects": "always-multiline",
"imports": "always-multiline",
"exports": "always-multiline",
"functions": "never"
}
]
}
}
Eslint for Drupal theme
The configuration for the Drupal
theme differs from the static site configuration, as it inherits .eslintrc.json
from the Drupal
core.
We can extend the configuration file from the Drupal
core with whatever rules we see fit, or that are adopted by the team. In my case, I print an error when using jQuery.once and add some small rules related to comma at the end of the line and the quotation marks. The list of Eslint
rules is huge, you can look it up in the documentation.
{
"extends": ["../../../core/.eslintrc.json"],
"globals": {
"context": true,
"settings": true,
"once": true
},
"plugins": ["prettier"],
"rules": {
"prettier/prettier": "error",
"quotes": ["error", "single"],
"comma-dangle": [
"error",
{
"arrays": "always-multiline",
"objects": "always-multiline",
"imports": "always-multiline",
"exports": "always-multiline",
"functions": "never"
}
],
"no-restricted-syntax": [
"error",
{
"selector": "MemberExpression[property.name = 'once']",
"message": "jQuery once is not allowed. Use native Drupal once implementation."
}
]
}
}
Eslint for legacy code in Drupal
This configuration is suitable for modules which nobody bothered to translate to ES6+ standard.
We inherit legacy .eslintrc.json
from Drupal core, and add rules as we see appropriate.
{
"extends": ["../../../core/.eslintrc.legacy.json"],
"env": {
"es6": false
}
}
I hope you don't have to write legacy JavaScript
anymore, because it's not a very enjoyable thing to do.
Have a good code!
References: