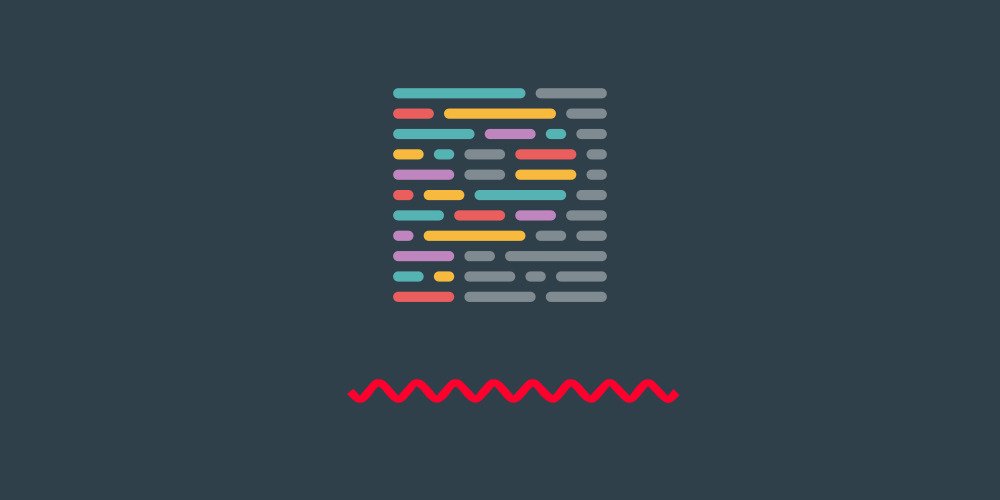
Prettier. How to set up in the right way
Prettier
will help uniformize code when working in a team and make it look nice with auto-formatting.
Installing Prettier
Install the latest version of Prettier
:
npm i -D prettier
Prettier features. Why do we need it?
With Prettier
we get the benefits:
- The programmer focuses on writing code rather than formatting it.
- The team writes uniformly formatted code.
- Prettier knows how to work with many popular languages.
- You can put
Prettier
on thepre-commit
hook to have the code formatted just before committing to Github/Gitlab.
The differences between Prettier and Linters
Linters (Stylelint, Eslint) perform static code analysis and report warnings and errors about the code quality.
Prettier
only does formatting.
Prettier configuration
Since Prettier
has its own sets of configurations for code formatting, there are often cases where a particular rule is unsuitable for the team or the developer.
In that case, you can change the configuration.
There is a file .prettierrc.json
for this (you can use other formats as well, more details here)
My .prettierrc.json
looks like this:
{
"semi": false,
"singleQuote": true,
"printWidth": 120,
"trailingComma": "es5",
"endOfLine": "lf", // lf — for Linux/Mac, crlf — for Windows
// For Nunjucks templates
"overrides": [
{
"files": ".njk",
"options": { "parser": "html" }
}
]
}
It must be said that the set of rules in the configuration is quite limited, and this was done by the developers consciously.
Ignoring 3rd party libraries
We don't need to format the 3rd party libraries and compiled code for production. You can use the file .prettierignore
to set up ignoring.
An example of my .prettierignore
file:
node_modules/
vendor/
dist/
Ignore formatting
To ignore line of code or a code block, write a comment with prettier-ignore
before it:
HTML:
<!-- prettier-ignore -->
CSS:
/* prettier-ignore */
JS/TS:
// prettier-ignore
JSX/TSX:
{/* prettier-ignore */}
Markdown:
<!-- prettier-ignore -->
You can also use for markdown:
<!-- prettier-ignore-start -->
Some text
<!-- prettier-ignore-end -->
To ignore individual files, use the .prettierignore
file.
Disable autoformatting of some file types in VSCode
I usually correct Prettier's behavior in Vscode
by disabling autoformatting for some file types.
Twig
— I subjectively don't like Prettier's formatting style in Twig
templates, so I turn it off.
Yaml
— autoformatting more than once led to the situation that *.yml
files do not pass validation in Drupal
because Prettier
"corrected" the length of the string. And as you know, in Yaml
indentation is crucial.
The VSCode
configuration looks like this:
{
"[html]": {
"editor.defaultFormatter": "vscode.html-language-features"
},
"[javascript]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[javascriptreact]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[typecript]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[typescriptreact]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[json]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[scss]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[pcss]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[vue]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[twig]": {
"editor.formatOnSave": false
},
"[yml]": {
"editor.formatOnSave": false
},
"[yaml]": {
"editor.formatOnSave": false
}
}
Format all project code at once
To format all the project code at once, execute the command in the console:
prettier --write "folder_name/**/*"
# In the current folder with subfolders
prettier --write .
Alternatively, you can add this script to the scripts
section of the package.json
file.
Outro
Bundlers like Vite
turn on Prettier
by default when you install an application like Vue
, and you don't have to worry about configuring it anymore.
In fact, you should set up Prettier
once and forget about formatting code by hand forever.
References: