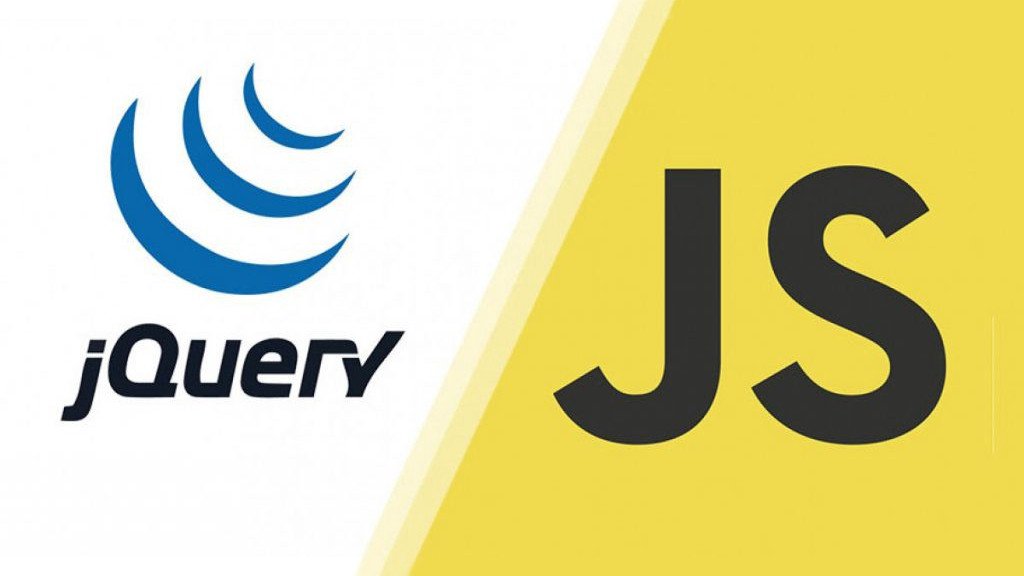
How to update Drupal Behavior code to vanilla JavaScript
Working on a Drupal theme, there are often situations for attaching various interactive JavaScript elements to a page. Since Drupal has quite a lot of Ajax calls (including Big Pipe
), it is often important to attach JavaScript in such a way that it is always triggered after Ajax rendered page elements. To do this we use Drupal.behavior
(in combination with once()
).
In the light of the global jQuery
abandonment in the frontend, we have to update the Drupal.behavior
code so that it uses vanilla JavaScript.
jQuery
mymodule.libraries.yml
myfeature:
js:
js/myfeature.js: {}
dependencies:
- core/drupal
- core/jquery
- core/jquery.once
myfeature.js
(function ($, Drupal) {
Drupal.behaviors.myfeature = {
attach(context) {
const $elements = $(context).find('[data-myfeature]').once('myfeature')
// `$elements` is always a jQuery object.
$elements.each(processingCallback)
},
}
function processingCallback(index, value) {
// some code...
}
})(jQuery, Drupal)
JavaScript
mymodule.libraries.yml
myfeature:
js:
js/myfeature.js: {}
dependencies:
- core/drupal
- core/once
myfeature.js
(function (Drupal, once) {
Drupal.behaviors.myfeature = {
attach(context) {
const elements = once('my-feature', '[data-my-feature]', context)
// `elements` is always an Array.
elements.forEach(processingCallback)
},
}
// The parameters are reversed in the callback between jQuery
// `.each` method and the native `.forEach` array method.
function processingCallback(value, index) {
// some code...
}
})(Drupal, once)
You can notice that the code has become more elegant and simpler.
References:
Last Updated: